Overview
Duty Planner is a desktop address book application used foradding MINDEF personnel, and assigning and handling duties for the personnel involed. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10kLoC.
Summary of contributions
-
Major enhancement: added accounts for different users, and other user functionalities.
-
What it does: Different personnel stored in the Duty Planner can log in. Accounts have 2 types: General and Admin, which can act differently for some commands. Some commands target the specific user.
-
Justification: This feature allows for commands that target the specific user (like swap duty, or edit own details). It also allows for commands like delete to be allowed for admins but not general users.
-
Highlights: The enhancement affects the Person model, and existing and future commands that may be added in the future. Many design alternatives were considered, and the implementation was difficult since it affects current commands, models and test cases.
-
-
Minor enhancement: edited the edit command to allow user to edit his own information (except NRIC and account type).
-
Code contributed: [Code]
-
Other contributions: Added functionality to Command Tests to allow testing based on UserTypes. Create test cases based on UserTypes and accounts for all previous commands.
-
Project management:
-
Helped in creating JAR file for team for version 1.3
-
-
Enhancements to existing features:
-
Helped other members to write CalendarView.fxml and CalendarView.java
-
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Log in
Logging into a User account
NRIC and passwords are case-sensitive.
Enter in NRIC and password into the top and bottom text areas respectively, press enter.
View all duties for current month: viewCurrent
Brings up calendar view, with all duties for the current month.
Format: viewCurrent
View all duties for next month: viewNext
Brings up calendar view, with all duties for the next month.
Format: viewNext
Adding a user : add
Add a user to the system with the corresponding NRIC, company, section, rank, name and contact number.
Format: add nr/NRIC c/COMPANY s/SECTION r/RANK n/NAME p/PHONE [t/TAG]
Each of the following fields entered by the user following each prefix are compulsory, and must adhere to the following format (Only the Tag field is optional):
Example:
-
add nr/S9012345L c/Echo s/2 r/CFC n/William Tan p/91234567 t/injured
Adds CFC William Tan in Echo Company Section 2 into the database.
Edit own details : edit
Edits user’s own details. Can edit one or more fields.
Format: edit [c/COMPANY] [s/SECTION] [r/RANK] [n/NAME] [p/PHONE] [t/TAG] [pw/PASSWORD]
Examples:
-
edit p/84523546 r/CPL
and
Edits the phone number and rank of the user to be `84523546CPL
respectively. -
edit c/Hotel t/ pw/pass
Edits the company of the user to beHotel
, clears all existing tags, and changes password to 'pass'.
Edit any user’s details : edit
Edits an existing user’s details in the personnel list.
Format: edit INDEX [nr/NRIC] [c/COMPANY] [s/SECTION] [r/RANK] [n/NAME] [p/PHONE] [t/TAG] [pw/PASSWORD] [u/A or G]
Examples:
-
edit 2 p/84523546 r/CPL u/A
Edits the phone number and rank of the 2nd person to be84523546
andCPL
respectively, and grants the person’s account administrator privileges. -
edit 1 c/Hotel t/ pw/pass
Edits the company of the 1st person to beHotel
, clears all existing tags, and changes password to 'pass'.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Logging in and account authority feature
Current Implementation
-
Note that in this segment, NRIC and Username may be used interchangeably, since the username is always the NRIC, unless using the default Admin account.
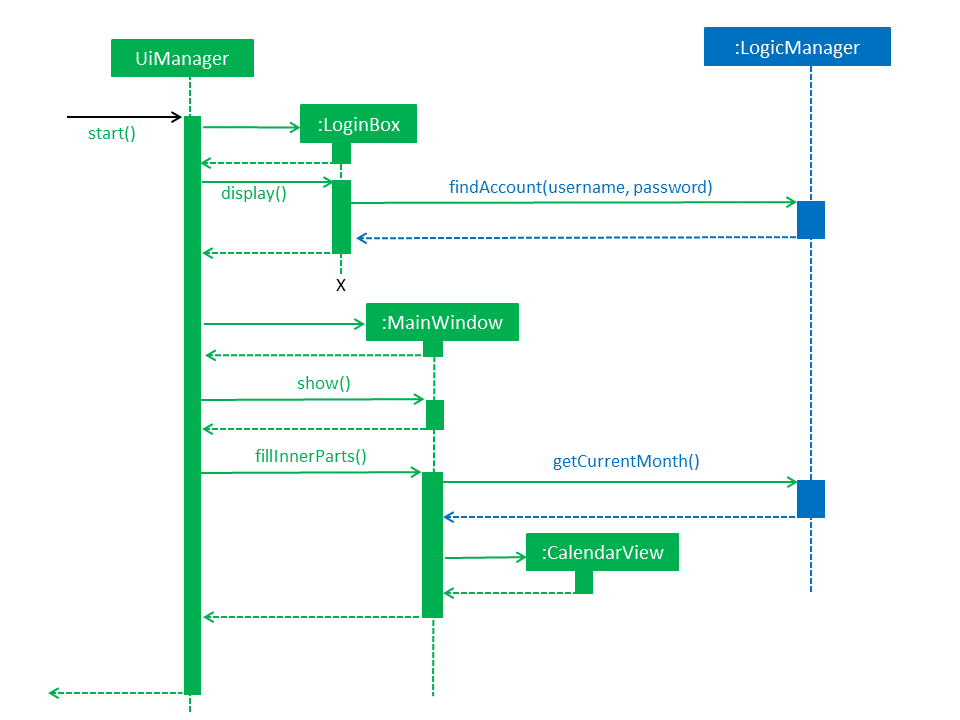
There are currently 2 UserTypes found in commons.core.index: UserType.ADMIN and UserType.General. Each NRIC account will be assigned to one of these user types that correspond to different authorities. Note that Admin type user accounts are not only have access to more commands, but some commands (such as edit) have more functionalities as well. The Command class implements AdminCommand and GeneralCommand interfaces.
Upon entering the username and password into the LoginBox, the LogicManager searches the list of personnel until it finds a personnel with the same username and (hashed) password. This returns the username and UserType of the account, and initializes the MainWindow with the username and Usertype as fields. The MainWindow then initializes the commandbox with these fields as well: the usertype so that the commands are executed using executeAdmin() or executeGeneral(), and the nric for several commands that require it to execute (for example, using the "Edit" command as a general account, since the account can only affect its own information.
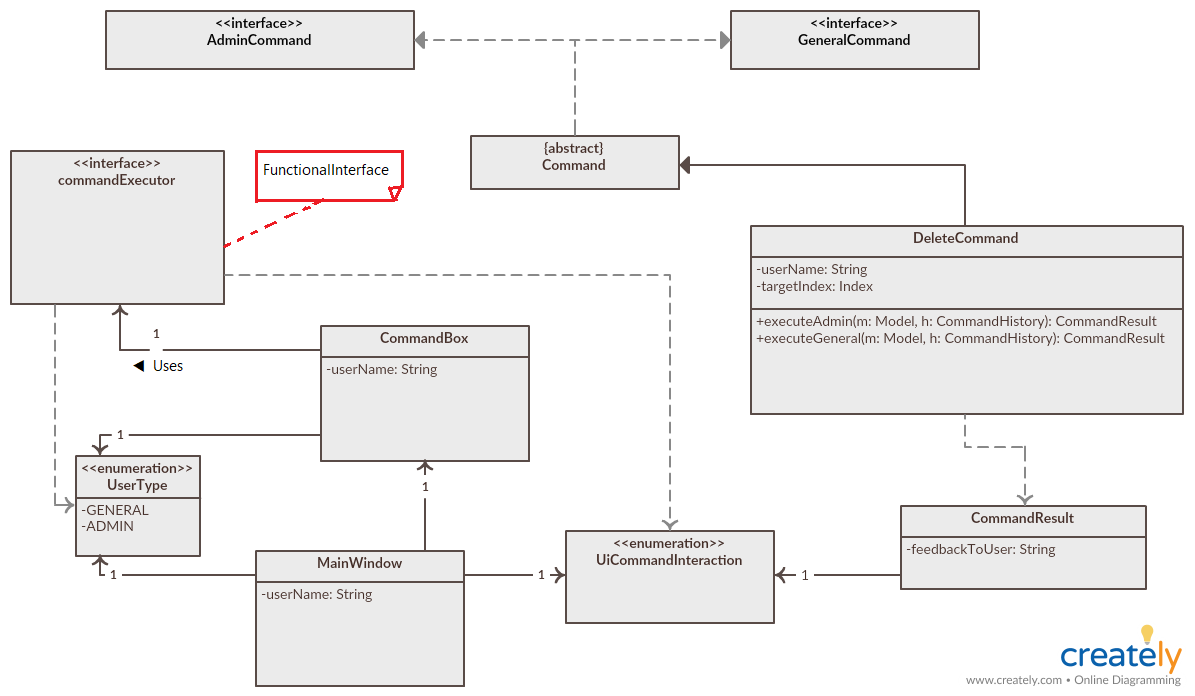
The execute Command in logic will decide to initialize the commands as either AdminCommand or GeneralCommand, to ensure the user does not have access to the wrong authority level.
Design Considerations
Aspect: handling logging in and UserType
-
Alternative 1 (current choice): LogIn box handles logic of finding account, MainWindow has UserType and NRIC field.
-
Pros: MainWindow initialized with a final UserType and NRIC field, which in turn creates a commandbox with a final UserType and NRIC field, which is safer for the commands, making sure the commands will be created as an AdminCommand or GeneralCommand correctly.
-
Cons: UI best not to handle logic, UserType or NRIC of user might be might be changed, but since the UserType and NRIC fields in the MainWindow are final, they cannot be changed. This might mess up some commands or functionalities that target the admin’s own account.
-
Implemented fix for cons: Login Box does not store Logic, but rather logic has a method to find the account given the NRIC and password, but LoginBox has a functional interface of the method as a field. To handle security concerns of the user changing his own NRIC or UserType, the program automatically exits once an admin account changes his own UserType or NRIC, or an admin deletes his own account.
-
-
Alternative 2: Logging in is now a command, where LoginCommand extends Command. After which the MainWindow creates the initializes the CommandBox with UserType and NRIC.
-
Pros: This is easier to implement, since the command functionality has already been set up. Logic operations now handled by Logic class, UserType and NRIC can be final attribute of CommandBox as well. After the admin changes his own UserType or NRIC, a new commandbox may be initialized with the new fields, instead of exiting the program.
-
Cons: Difficult to hide the password text this way, as we have to change how the command box textArea functions to hide password text. We also have to change the command in such a way that the logger does not save the command, since we do not want the the username and password being shown in the logger.
-
Aspect: handling commands that require UI changes
For example, the ExitCommand, HelpCommand, ListCommand, ViewNextCommand.
-
Alternative 1 (current choice): An enum type (UiCommandInteraction.java) exists for each kind of possible UI change in the program. For example, there is a UiCommandInteraction.EXIT and UiCOmmandInteraction.CALENDAR_CURRENT. When ViewCurrentCommand is executed, a CommandResult will have a field that contains the enum type, and the Ui change will be executed based on this enum type.
-
Pros: Easy to implement, especially for this iteration of Duty Planner, where there aren’t many UI changes.
-
Cons: More tedious to implement if there are commands that use more UI changes, due to the possible number of permutations. For example, if you want a command to update the calendar to the next month, but also show the personnel list view, while bringing up a help window. The switch case can get very huge quickly the more commands there are.
-
-
Alternative 2 (previous iteration of AB4): CommandResult has boolean fields "exit" and "showHelp", as well as methods "isExit()" and "isShowHelp()". After executing the command, the CommandResult is checked for each off these fields, and the relevant UI change is executed for each of the boolean fields.
-
Pros: Easy to implement, does not have the downside of the previous alternative, since each field only needs to be checked once, so the number of checks only grows linearly with the possible number of UI changes.
-
Cons: Very tedious and more prone to error. For example, in AB4, the ExitCommand returns new CommandResult(message, false, true). If there are many fields like DutyPlanner 1.4, it will be CommandResult(message, false, true, false, false, false), which is both tedious for most commands, and prone to error in the long run, especially if even more UI changes are implemented.
-
Possible fix for cons: Similar to how a PersonBuilder is used to AB4, testcases, create a CommandResultBuilder class, with UI fields being the same as Command Result, but all UI fields false by default. To create a command result that shows help and shows the list, simply use: new CommandResultBuilder().withHelp().withList().build(). This should reduce tediousness and results in less errors.
-
PROJECT: PowerPointLabs
{Optionally, you may include other projects in your portfolio.}