Overview
MINDEF Duty Planner is a desktop application used to help military units in the Singapore Armed Forces organise and plan monthly duties for their servicemen. It has various features and functions for administrator (i.e. commander) as well as the duty personnel (i.e. servicemen). The user interacts with the application using a Command Line Interface (CLI) with a Graphic User Interface (GUI) created with JavaFX. It is written in Java, and has about 10kLoC.
The Duty Planner is modified and upgraded from SE-EDU Address Book 4.
Summary of contributions
-
Major enhancement: Creation of the duty package module
-
What it does: The duty package consists of classes (i.e. Duty, DutyMonth, DutySettings, DutyStorage) responsible for setting up the duties of a month and assigning them to persons, as well as contains vital functions needed for the duty features to be implemented.
-
Duty
: A class that characterizes the attributes of a duty including the exact date, points awarded, manpower needed and a list of person eventually being assigned to that duty. -
DutyMonth
: Represents a month in the calendar and is the main driver class behind the scheduling of duties. It instantiates all duties and assigns each duty to persons. Stores vital information such as the list of scheduled duties and the blocked dates of each person. -
DutySettings
: Contains information about the manpower needed and points assigned to duties based the day of the week. Enable modification to the the manpower needed and points assigned to each duty based on the day of the week. -
DutyStorage
: Accumulates and stores the duty points and duty records of all the persons.
-
-
Justification: The duty package provides the backend support for all duty-related features and commands.
-
Highlights: The package contain functions assessed by many commands (i.e. BlockDateCommmand, ScheduleCommand, DutySettingsCommand, ViewCommand, PointsCommand, RewardCommand, PenalizeCommand).
-
Credits: {Teammate Yoon Ki Hyun for working together on the DutyMonth and Duty}
-
-
Major enhancement:
ScheduleCommand
andConfirmScheduleCommand
-
What it does: The schedule command that invokes the schedule algorithm to generate a viable duty allocation for the upcoming month. The confirm command finalizes the schedule and updates the existing duty points of each person.
-
Justification: Enable duties to be created and scheduled.
-
Highlights: The main feature of the application.
-
-
Major enhancement:
SettingsCommand
-
What it does: Enable the administrator to adjust the desired manpower for duties based on the day of the week. Enable the administrator to adjust the points to be awarded for doing duties based on the duty of the week.
-
Justification: Allows the administrator to have flexibility to change the duty allocation system.
-
Highlights: Need to ensure that the scheduling algorithm is compatible with adjustable duty points and duty capacities.
-
-
Major enhancement:
PointsCommand
-
What it does: Enable the administrator to view duty points and duty records of all persons.
-
Justification: Allows more duty-related information to be viewed.
-
-
Major enhancement:
RewardCommand
andPenalizeCommand
-
What it does: Enable the administrator to reward and penalize points from a person or a list of persons.
-
Justification: Allows the administrator the autonomy to manually update duty points.
-
Highlight: Enabled us to better test the effectiveness of the scheduling algorithm in obscure situations (i.e. one person having points significantly higher or lower points than the rest).
-
-
Minor enhancement: Storage for
DutyStorage
—JsonAdaptedDutyStorage
-
What it does: Ensures that duty points and duty records are saved in the system when the application closes.
-
Justification: This allows users to open the application with previously saved duty information instead of resetting each time the application reopens.
-
-
Minor enhancement:
DateUtil
Common-
What it does: A class that contains static methods to assist in validation of date parameters and other date related functions.
-
Justification: Contains commonly used functions that aids the developers and prevent duplicate codes.
-
-
Code contributed: [Code]
-
Project management:
-
Managed releases
v1.1
-
-
Documentation:
-
Major updates to the User Guide:
https://github.com/yjpan47/main/blob/master/docs/UserGuide.adoc -
Updated Developer Guide to include Duty Package:
https://github.com/yjpan47/main/blob/master/docs/DeveloperGuide.adoc
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
View duty points : points
Displays the duty points accumulated by each person. Additional records of each person (i.e. duties allocated, points rewarded, points penalized) can also be viewed.
Format: points [INDEX]
Calling the command without index (points ) provides a list of all persons and their accumulated duty points while calling the command with index (points INDEX ) provides the accumulated duty points of an individual person and his record (i.e. duties, points rewarded, points penalized).
|
Examples:
-
points 2
Retrieves the duty points of the 2nd person on the contact list, as well as his records with information such as duties allocated, points rewards and points penalized. -
points
Displays a list of all persons and their respective duty points.
Reward points : reward
Manually reward duty points to a person or a list of persons.
Format: reward i/INDEX [INDEX] [INDEX] … p/POINTS
-
Points rewarded can range from 1 to 100 for each command call.
Examples:
-
reward i/1 2 4 5 p/20
Rewards 20 points each to the 1st, 2nd, 4th and 5th person on the contact list. -
reward i/3 p/4
Rewards 4 points to the 3th person on the contact list.
Penalize points : penalize
Manually penalize duty points for a person or a list of persons.
Format: penalize i/INDEX [INDEX] [INDEX] … p/POINTS
-
Points penalized can range from 1 to 100 for each command call.
-
The duty points of a person can fall to less than zero.
Examples:
-
penalize i/1 2 4 5 p/20
Penalize 20 points each for the 1st, 2nd, 4th and 5th person on the contact list. -
penalize i/3 p/4
Penalize 4 points for the 3th person on the contact list.
View and edit duty settings (points and manpower needed for each day of the week) : settings
View and edit duty settings. Duty settings refer to the manpower needed and points rewarded for each duty based on the day of the week (e.g. Sunday, Monday, etc)
Format: settings [d/DAYOFWEEK m/MANPOWER p/POINTS]
-
DAYOFWEEK
: Mon / Tue / Wed / Thur / Fri / Sat / Sun (full spelling e.g. Monday, Tuesday etc. works as well) -
MANPOWER
: Number ranging from 1 to 10 -
POINTS
: Number ranging from 1 to 100
Calling the command settings alone displays the current duty settings while calling the command settings with the day of the week, manpower and points enables the editing of the duty settings.
|
The updated duty settings will only take effect the next time a schedule is confirmed. If schedule for next month has already been confirmed, the confirmed schedule will still follow the specifications of the previous duty settings. |
Examples:
-
settings
Displays the duty settings - for each day of the week, the manpower needed and points to be rewarded. -
settings d/sun m/3 p/4
Duty settings edited. Sunday duties now require 3 persons and 4 points will be rewarded to each person assigned a Sunday duty. -
settings d/monday m/2 p/3
Duty settings edited. Monday duties now require 2 persons and 3 points will be rewarded to each person assigned a Monday duty.
Schedule duties : schedule
Creates a viable duty schedule for the upcoming month. The scheduling algorithm takes into account the manpower needs of each duty day, blocked dates of each person, the current duty points of each person and points rewarded for each duty to generate a fair schedule.
Format: schedule
The algorithm is optimized such that a person with low duty points will be allocated to duties which rewards high points and possibly be given more duties. It automatically attempts to balance out the points of each person after scheduling.
The command schedule merely provides a viable duty schedule and does not confirm the schedule to the calendar. Duty points have also yet been updated. The schedule generated is not deterministic and calling schedule again will regenerate a new duty schedule.
|
Once a satisfactory duty schedule is generated, the administrator can go ahead to confirm the duty schedule. (See 4.4.10 Confirm a schedule)
Calling schedule
after next month’s duties have already been confirm will only display the confirmed duty schedule.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Duty Package
Duty Month
DutyMonth
class represents all duty-related information for a particular month. It contains the following key information:
-
The blocked days of every person for duties in the month.
-
A list of scheduled duties implemented as a
Duty
object list-
At the construction of the
DutyMonth
, the list is empty. -
When the
schedule
method is called,Duty
objects are be instantiated and appended to the list. The list will contain the same number ofDuty
objects as the number of days in the month.
-
-
A boolean
confirm
state — denoting whether the duty allocation for the month is confimed.
DutyMonth
class contains the following key operations:
-
DutyMonth#addBlockedDay(person, day)
— adds a blocked day to a particular person, indicating that the person cannot be assigned duty on that particular day of the month. -
DutyMonth#removeBlockedDays(person)
— remove all blocked days for a particular person, indicating that the person can now be assigned duty on any day of the month. -
DutyMonth#schedule(personList, dutySettings, dutyStorage)
— invokes the scheduling alogorithm. Generate all duties for the particular month and assign person from personList to the duties. -
DutyMonth#confirm()
— sets the state of theDutyMonth
to confirmed. Duty allocation is finalized.
Duty
Duty
class represents a duty for a day. It contains the following information:
-
List of
Person
allocated to the duty. -
Capacity — number of persons needed for the duty.
-
Points — number of points to be rewarded to each person assigned to the duty.
Duty Storage
DutyMonth
class stores the accumulated duty points and duty records of every person. It has the following key operations:
-
DutyStorage#update(dutieslist)
— updates the duty points and records from a list of scheduled duties. Used when schedules are confirmed and finalized. -
DutyStorage#undo(dutieslist)
— restore to previous state of duty points and records. Used when previous confirmed duty schedule has to be undone. -
DutyStorage#reward(person, point)
— adds duty points to a particular person. -
DutyStorage#penalize(person, point)
— deducts duty points from a particular person.
Duty Settings
DutySettings
class is an attribute under UserPrefs
. It stores information regarding the manpower needed and duty points to be rewarded for each duty based on the day of the week.
It is accessed when duties are generated to ensure the appropriate attributes (capacity and points) given to each duty.
Schedule Command
The schedule
command is called by the admin to generate a viable duty schedule for the upcoming month.
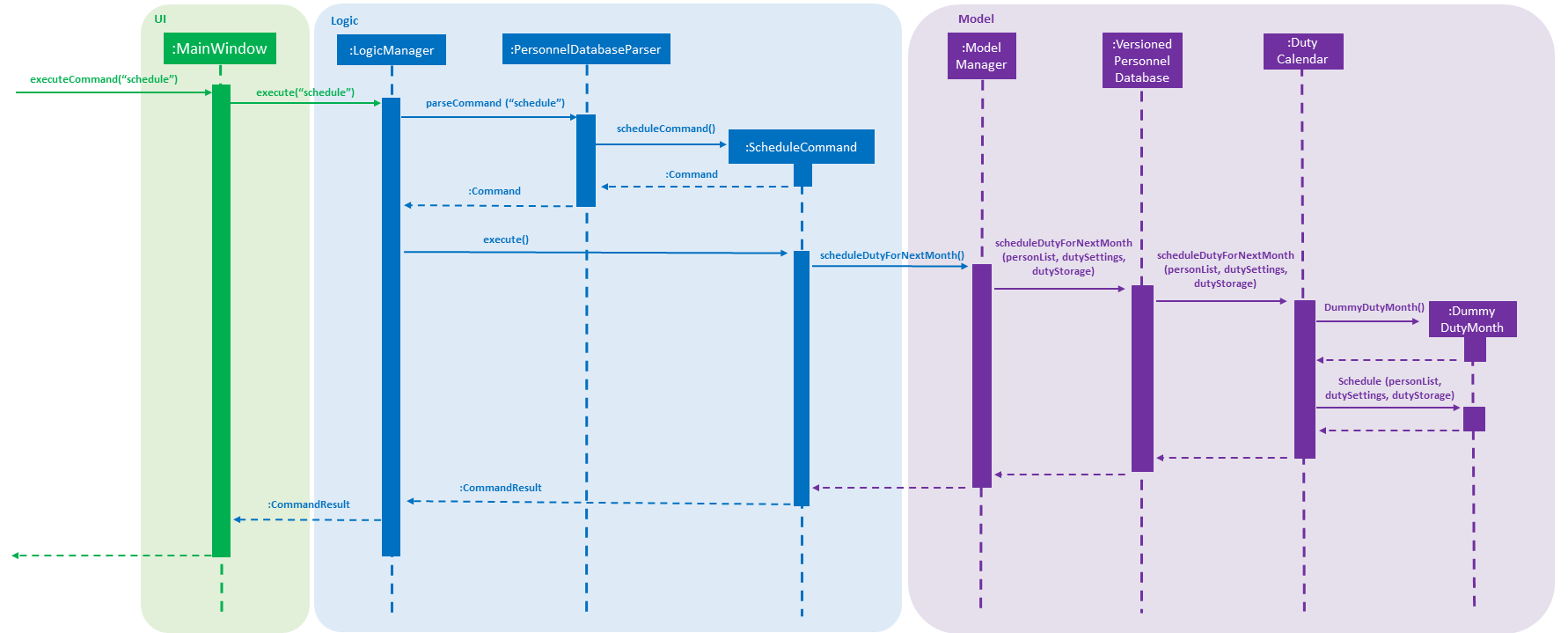
The sequence diagram above shows how the schedule
operation works.
It is worth noting that schedule
operation does not finalize the duty schedule for next month. Therefore, dutyCalendar
has to instantiates a new dummyNextMonth
for the operation.
Subsequent calling of the confirm
operation will allow the nextMonth
object to point to the latest dummyNextMonth
, and invoke dutyStorage
to update duty points and records.
Schedule Algorithm
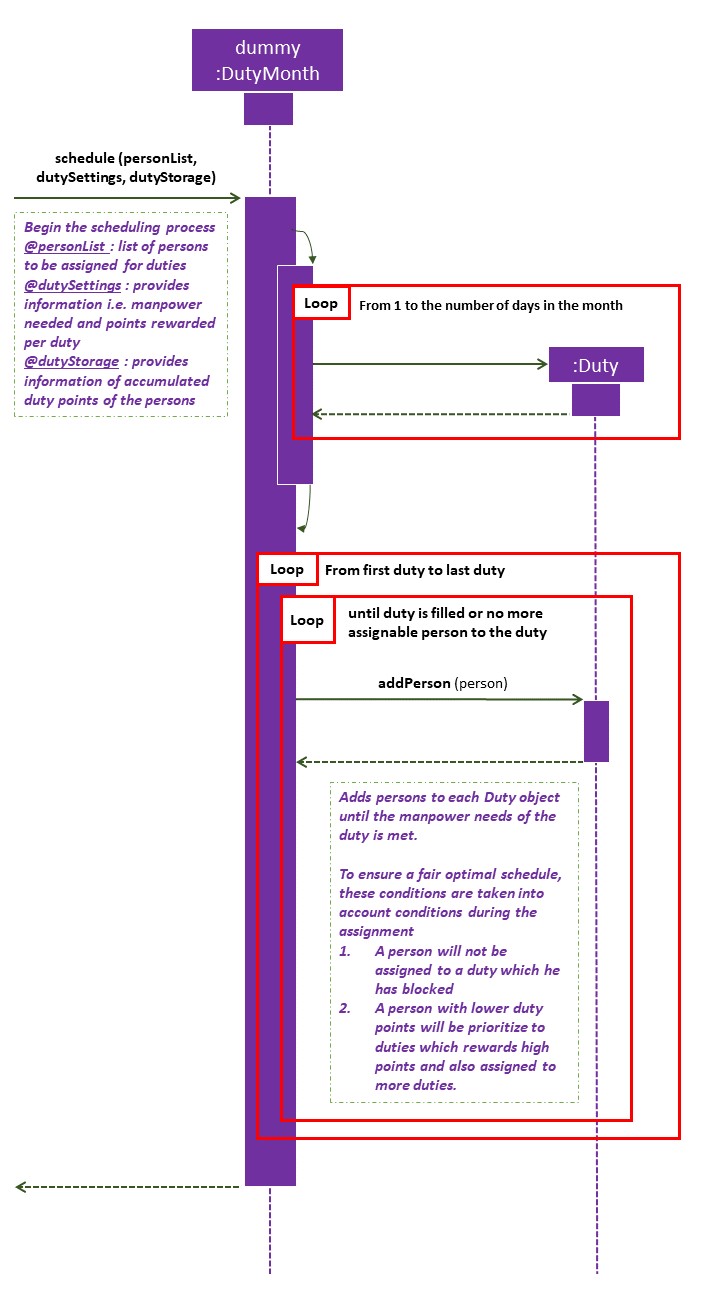
The sequence diagram above provides a simplified illustration of the schedule
method in DutyMonth
.
To ensure a system where duties are allocated fairly,
-
We employ a point system where each person can gain points by doing duties.
-
The points awarded are cumulative — can be carried over to the next month. Duty points accumulated are stored in
DutyStorage
. -
Each duty is worth a certain number of points and a person gains that amount of points by doing that duty. The points allocated to each duty is retrieved from
DutySettings
. -
Before the
schedule
command is called, each person has the opportunity to indicate the days he are unavailable for duty.
Approach
-
Generate a list of
Duty
objects sorted by their points in descending order. -
Generate a priority queue of all
Person
objects, queued based on their accumulated duty points with the lowest at the head of the queue. -
Iterate through the list of
Duty
objects. At each iteration,-
Poll the priority queue of
Person
continuously until an instance of a compatible duty-person pairing. -
A compatible pairing consists of conditions such as the
Person
not having blocked the day of theDuty
and theDuty
does not already contain thePerson
. -
Iteration continues after the
Duty
is filled or priority queue ofPerson
becomes empty (cannot find compatiblePerson
).
-
-
The algorithm ensures that a person with low accumulated duty points will be assigned to duties that rewards higher points and assigned to more duties. This allows the scheduler to automatically balance the duty points of each person by the end of the month.
Design Considerations
Aspect: Storage of Duty issues
-
Alternative 1 (current choice):
Duty
class also plays the role ofDutyDate
class-
Pros: one less layer for simplicity
-
Cons: less flexible for extension of the project, hard to ensure that each
DutyMonth
contains the correct number ofDuty
objects
-
-
Alternative 2: create a separate
DutyDate
class that containsDuty
as an attribute-
Pros: Easy indexing and reference for UI and other purposes
-
Cons:
DutyDate
class does not have other roles, can be redundant
-
Aspect: Storing duty points of each person
-
Alternative 1 (current choice): Stored in external
DutyStorage
object with HashMap attribute.-
Pros: Reduce number of dependencies —
Person
class is completely freed from any duty information. Duty package operates independently. -
Cons: A lot of inefficiencies when querying duty points.
-
-
Alternative 2: Enable
Person
class to contain duty related information. For example, adding a duty point attribute toPerson
.-
Pros: Simple and efficient indexing and referencing.
-
Cons: Increase level of dependencies. Could potentially result in circular dependencies between
Duty
andPerson
.
-
Schedule duties for next month
-
Schedule duties for the next month.
-
Prerequisites: Multiple persons in the list.
-
Test case:
schedule
Expected: The schedule for the next month should be generated.
Scroll down to see the duty roster and points to be awarded to the persons.
-
Confirm schedule for next month
-
Confirm a schedule for next month.
-
Prerequisites:
schedule
has just been called. -
Test case:
confirm
Expected: Schedule has been successfully confirmed.
Scroll down to see the duty roster and total points accumulated for the persons.
CallviewNext
to see the confirmed schedule on the calendar.
-
Reward points to persons
-
Rewards points to a person or a list of persons
-
Prerequisites: Multiple persons in the list. Call
points
to view current duty points of the persons. -
Test case:
reward i/1 p/10
Expected: 1st person on the contact list has his duty points increase by 10. Callpoints
again to verify. -
Test case:
reward i/1 2 4 p/30
Expected: 1st, 3nd and 4th persons on the contact list have their duty points increase by 30 each. Callpoints
again to verify.
-
Penalize points to persons
-
Penalize points to a person or a list of persons
-
Prerequisites: Multiple persons in the list. Call
points
to view current duty points of the persons. -
Test case:
penalize i/1 p/15
Expected: 1st person on the contact list has his duty points decrease by 15. Callpoints
again to verify. -
Test case:
penalize i/2 3 p/5
Expected: 2nd and 3rd persons on the contact list have their duty points decrease by 5 each. Callpoints
again to verify.
-
Change duty settings
-
Change duty settings
-
Prerequisites: Multiple persons in the list. Duty schedule for next month not confirmed. Call
schedule
to get a duty allocation. When viewing the duty roster result from the scheduling, take note of the points awarded by each duty and the number of persons allocated to each duty based on which day of the week it is. -
Test case:
settings
Expected: Duty settings get displayed, indicating the manpower needed and points rewarded for duties based on which day of the week the duty falls on. These numbers should tally with the observations from callingschedule
previously. -
Test case:
settings d/sun p/10 m/5
Expected: Sunday duties now require 5 persons and worth 10 points each. Callschedule
again and observe the duty roster result. The new duty roster should provide information that tallies with the changed duty settings
-